Joined on over 1 year ago
Self-study Computer Science
Have you been a professional self-taught software engineer and sensed a gap in your knowledge? It's possible that you're feeling the effects of lacking a formal CS education. Fortunately, there's premium content available to bridge that gap.
Don’t be a boilerplate programmer. Instead, build tools for users and other programmers. Take historical note of textile and steel industries: do you want to build machines and tools, or do you want to operate those machines?
— Ras Bodik at the start of his compilers course
TL;DR:
Study all eight subjects below, in roughly the presented order, using both the suggested textbook and video lecture series. Aim for 100-200 hours of study of each topic, then revisit favorites throughout your career 🚀.
Why learn computer science?
There are 2 types of software engineer: those who understand computer science well enough to do challenging, innovative work, and those who just get by because they’re familiar with a few high level tools.
Both call themselves software engineers, and both tend to earn similar salaries in their early careers. But Type 1 engineers progress toward more fulfilling and well-remunerated work over time, whether that’s valuable commercial work or breakthrough open-source projects, technical leadership or high-quality individual contributions.
Type 1 engineers find ways to learn computer science in depth, whether through conventional means or by relentlessly learning throughout their careers. Type 2 engineers typically stay at the surface, learning specific tools and technologies rather than their underlying foundations, only picking up new skills when the winds of technical fashion change.
Currently, the number of people entering the industry is rapidly increasing, while the number of CS grads is relatively static. This oversupply of Type 2 engineers is starting to reduce their employment opportunities and keep them out of the industry’s more fulfilling work. Whether you’re striving to become a Type 1 engineer or simply looking for more job security, learning computer science is the only reliable path.
Too much content?
If the idea of self-studying 8 topics over multiple years feels overwhelming, you should focus on just two books: Computer Systems: A Programmer's Perspective and Designing Data-Intensive Applications. These two books provide incredibly high return on time invested, particularly for self-taught engineers and bootcamp grads working on networked applications. They may also serve as a "gateway drug" for the other topics and resources listed above.
FAQ
Who is the target audience for this guide?
A self-taught software engineer, bootcamp grad or precocious high school student, or a college student looking to supplement your formal education with some self-study. The question of when to embark upon this journey is an entirely personal one, but most people tend to benefit from having some professional experience before diving too deep into CS theory. For instance, most love learning about database systems if they have already worked with databases professionally, or about computer networking if they’ve worked on a web project or two.
How strict is the suggested sequencing?
Realistically, all of these subjects have a significant amount of overlap, and refer to one another cyclically.
As such, our suggested sequencing is mostly there to help you just get started… if you have a compelling reason to prefer a different sequence, then go for it. The most significant “pre-requisites” are: computer architecture before operating systems or databases, and networking and operating systems before distributed systems.
What about language X?
Learning a particular programming language is on a totally different plane to learning about an area of computer science — learning a language is much easier and much less valuable. If you already know a couple of languages, we strongly suggest simply following our guide and fitting language acquisition in the gaps, or leaving it for afterwards. If you’ve learned programming well, and especially if you have learned compilers, it should take you little more than a weekend to learn the essentials of a new language, after which you can learn about the libraries/tooling/ecosystem on the job.
What about trendy technology X?
No single technology is important enough that learning to use it should be a core part of your education. On the other hand, it’s great that you’re excited to learn about that thing. The trick is to work backwards from the particular technology to the underlying field or concept, and learn that in depth before seeing how your trendy technology fits into the bigger picture.
This is mostly based on teachyourselfcs with a few modifications I made based on my personal experience completing it. You might also want to check out OSSU.
Sub actions
Programming
This course provides a foundation for understanding how to use language constructs and how to design good programs. By using various languages, you'll go beyond just one language's syntax. Functional programming is important for writing solid, reusable, and straightforward programs. By the end of the course, you'll be comfortable with different languages and approaches, and you'll have the skills to choose the right one for the task.
Programming Languages, Part A
Programming Languages, Part B
Programming Languages, Part C
Computer Architecture
Ever wondered how the code you've written actually works? How are programs executed? What does executing a program even mean? Who's responsible for executing it: the OS, CPU, or both? How are variables stored in RAM? What are the stack and heap? How do int and float types differ? How can we run thousands of programs with only 6 cores? What are those L1 and L2 caches you've heard about? Most of these mysteries will be unraveled upon completing this section.
Build a Modern Computer from First Principles: From Nand to Tetris
Best way to understand how something works is to just build it yourself. You will build a CPU, RAM an your own machine language (assembly) and put it together to build your very own computer in code! The course has two parts. Completing just the first part is sufficient.
Prerequisite for CS:APP - Learn C
C is the lingua franca of computer science. It's straightforward yet potent. C is one of the languages that most closely align with assembly language.
By learning C, you will be able to understand and visualize the inner workings of computer systems (like allocation and memory management), their architecture and the overall concepts that drive programming. As a programming language, C also allows you to write more complex and comprehensive programs.
CS:APP

If you've reached this point, you possess a basic understanding of how the CPU, RAM, and machine language work. Let's delve deeper. This book explains how modern computer systems, specifically x86-64, operate. Remember the assembly language that seemed daunting? After finishing CS:APP, it will become much more digestible.
This book demystifies computing. It's challenging, so take your time. Absorb each chapter thoroughly. The labs are demanding, and while you may be tempted to seek answers online, resist. The satisfaction derived from tackling them yourself is immense. Attempt every exercise.
Data Structures and Algorithms
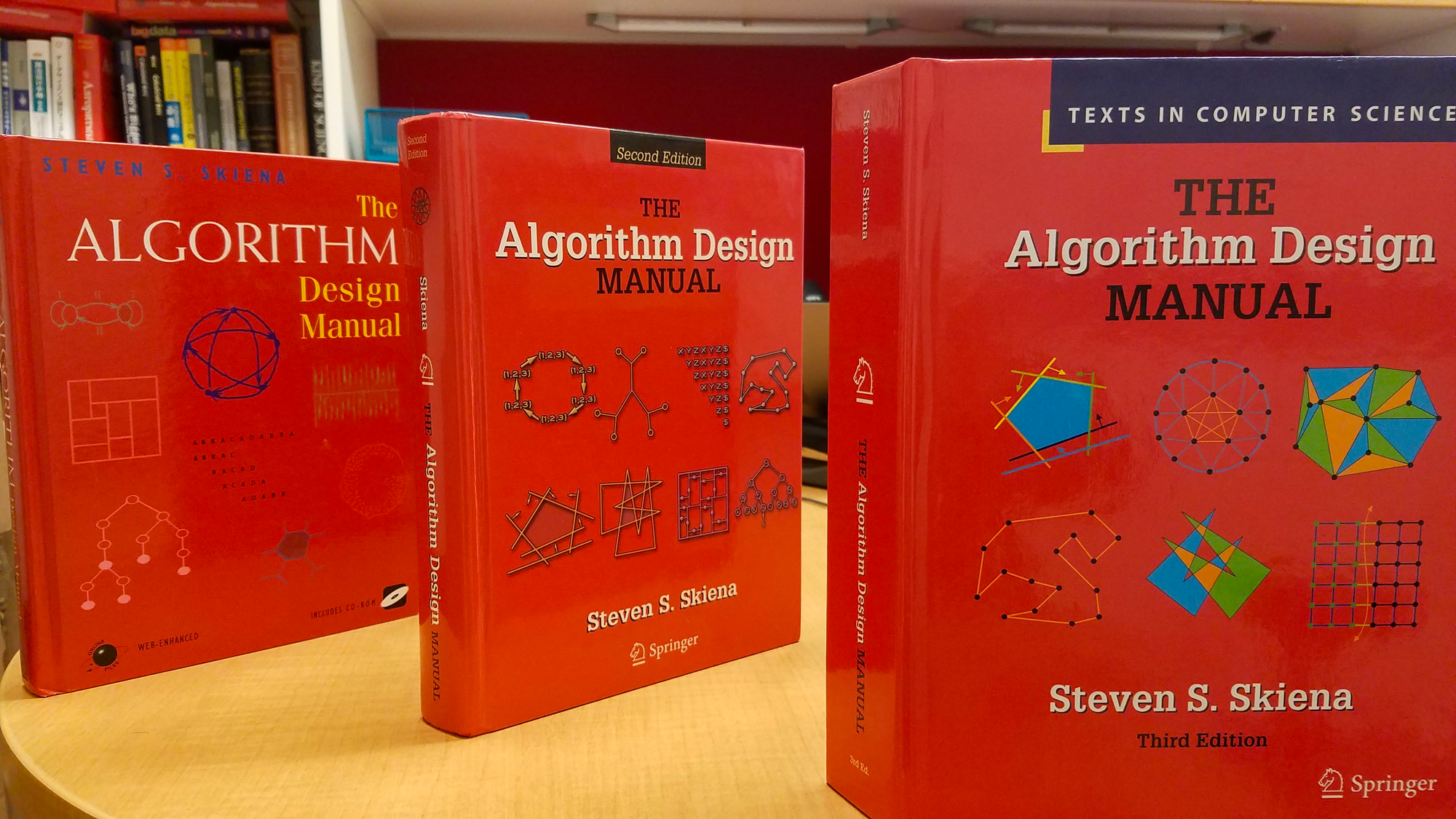
Algorithms and data structures is one of the most empowering aspects of a computer science education. This is also a great place to train one’s general problem-solving abilities, which will pay off in every other area of study. For practice, solve 5 medium leeetcode problems after each chapter
Having a firm understanding of how data structures laid out in memory enhances your understanding of speed and memory complexity. This lets you implement data structures behind the algorithms without any smokescreen going on. If you understand data structures with C, you will basically understand how its implemented in other languages.
That's why I prefer Steven Skienna's book. It uses C, and isn't proof-heavy.
Operating Systems
Operating systems might be the most magical software ever written. It is the bridge between software applications and computer hardware. Understanding how it works provides insight into the foundational principles of computer science and how computers function at a basic level.
Operating Systems: Three Easy Pieces
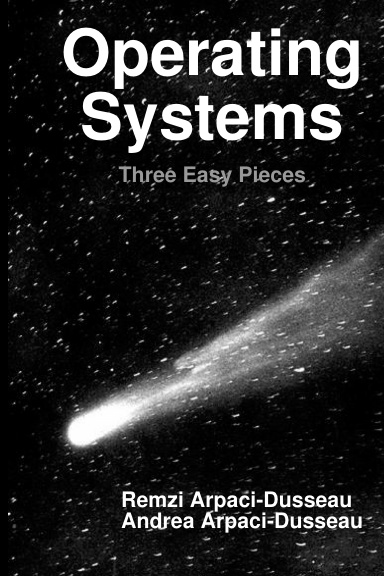
The book is freely available online, as well as the lectures.
The lecturer excels in simplifying complex topics. He clearly enjoys teaching. I absolutely loved his video lectures. Easily one of my favorites.
OSSU has an amazing guide for how to go through the lectures, I'll just link it here. Ensure you've completed the "From Nand to Tetris Part 1" and "CS:APP" before reading this book. Otherwise, the content might be puzzling.
Optional: The Design and Implementation of the FreeBSD Operating System

If you've enjoyed operating systems as much as me, you probably now wondering how to write your own operating system from scratch. I'd recommend going through this gem as you will understand the concepts behind a modern unix OS, bolstering your confidence in creating your OS.
Book
Computer Networking

Since the dawn of the internet, computer networking has been one the most important subjects for software engineers.
Given that so much of software engineering is on web servers and clients, one of the most immediately valuable areas of computer science is computer networking. After you study networking find that you will finally understand terms, concepts and protocols you've been surrounded by for years.
Databases
Any software or web application that needs to store user data, transactional data, or any other kind of data relies on a database. Knowing how databases work is crucial for backend development. However it takes more work to self-learn about database systems than it does with most other topics.
Prerequisite: C++
The CMU course uses C++ for labs. A decent grasp of C++ is required to succeed in the labs. If you aim to acquire just enough C++ to complete the course, consider the C++ bootcamp crafted by the course creators. Navigate the code and peruse the comments
For a more rigorous learning experience I'd suggest doing learncpp. It assumes zero prior programming experience.
Introduction To Databases - CMU
This course is a great intro to understanding the inner mechanism of a relational database.
Topics include data models (relational, document, key/value), storage models (n-ary, decomposition), query languages (SQL, stored procedures), storage architectures (heaps, log-structured), indexing (order preserving trees, hash tables), transaction processing (ACID, concurrency control), recovery (logging, checkpoints), query processing (joins, sorting, aggregation, optimization), and parallel architectures (multi-core, distributed). Case studies on open-source and commercial database systems are used to illustrate these techniques and trade-offs.
Languages and Compilers

You may know how to code in one or more programming languages.
But do you know how to create or design one? That's what you will learn by studying programming languages and compilers.
The recommended introductory book is called Crafting Interpreters.
Distributed Systems
Distributed systems is the holy grail for tech companies and understanding it is a prerequisite for passing system design interviews. Have you ever wondered how replicas work? How big tech serves millions of people? It's time to remove yet another magic.
Read: Understanding Distributed Systems
Do the MIT course
Read: Designing Data Intensive Applications
Understanding Distributed Systems: What every developer should know about large distributed applications
MIT 6.824
In this course, you will implement a consensus algorithm and a fault-tolerant KV service built upon it.
Begin by reading the preparatory papers, followed by watching the video lecture. Reading the papers is CRUCIAL. Do not skip them.
The labs will be quite challenging to implement. Ensure you set up a proper logging system; otherwise, completing your labs will become an insurmountable task.
Refer this post whenever you get stuck.
Designing Data-Intensive Applications
Comments
No comments yet
Would you like to make the first comment?